Delegation
We need inheritance of more than one activity in base activity. It is necessary to combine them, but there is no multiple inheritance in kotlin. What are we gonna do?
Delegation is a design pattern. we use this pattern with by. When we use by lazy or by viewModel, we use the concept of delegation.
The base activity doesn't know exactly what's inside the class. Therefore, responsibility is not very clean when inheritance is used. So we can use delegation in this case.
Delegation Is Not Inheritance! in Kotlin, we can delegate not only to interfaces but to separate properties as well.
Why do we use delegation?
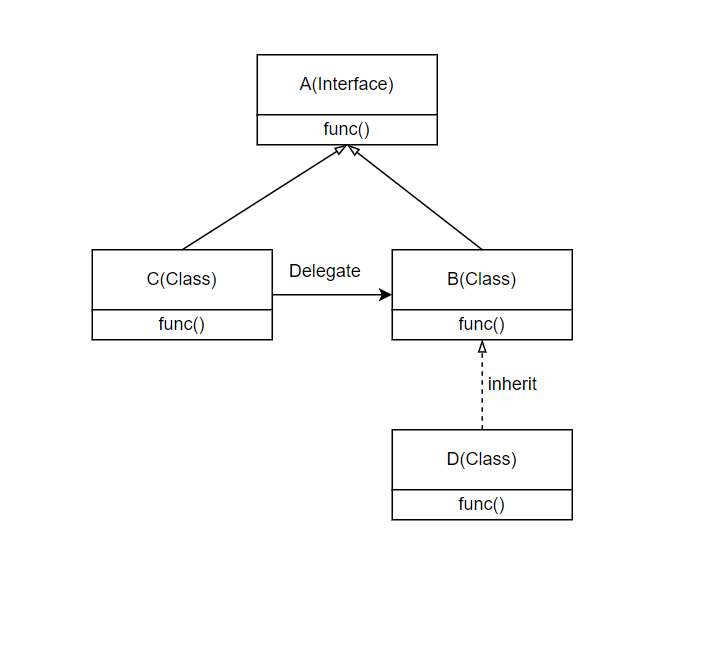
Code Example
interface Nameable{
var name : String
}
class AtillaName : Nameable{
override var name:String ="Atilla"
}
class LongDistanceRunner: Runnable{
override fun run() {
println("too long ago")
}
}
class People(name:Nameable,runnable: Runnable): Nameable by name, Runnable by runnable
fun main(args: Array) {
val person = People(AtillaName(),LongDistanceRunner())
println(person.name)
person.run()
}
Output:
Atilla
too long ago
INHERITANCE VS. DELEGATION
inheritance
open class RealPrinter{ //open keyword is necessary for inheritance in kotlin unlike java
open fun print(){
println("printing data")
}
}
class Printer : RealPrinter() {
override fun print(){
super.print() //parent's print func.
}
}
fun main(args: Array) {
val printer = Printer()
printer.print()
}
Output:
printing data
delegation
open class RealPrinter{ //open keyword is necessary for inheritance in kotlin unlike java
open fun print(){
println("printing data")
}
}
class Printer{
val p = RealPrinter()
fun print(){
p.print()
}
}
fun main(args: Array) {
val printer = Printer()
printer.print()
}
Output:
printing data
When to use what?
-> Some good resources on the subject: